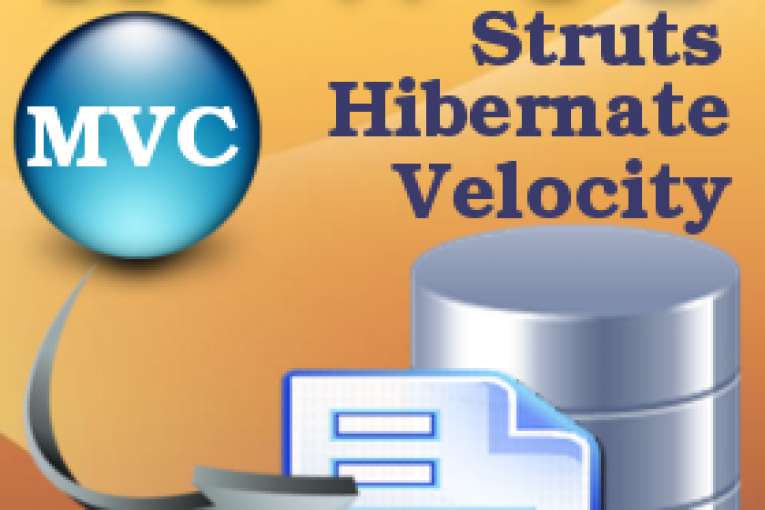
In this article I’ve provided a basic tutorial to create a java web-project skeleton based on the MVC (Model View Controller) design pattern. This pattern is used to separate and stratify the logic of a web application.
– Model represents an object or java POJO carrying data. It can also have logic to update controller if its data changes.
– View represents the visualization of the data that model contains.
– Controller acts on both Model and view. It controls the data flow into model object and updates the view whenever data changes. It keeps View and Model separate.
I’ve based this tutorial on the following three java technologies:
– Apache Struts: the MVC framework of the Apache Foundation
– Hibernate: the ORM framework for the persistence layer
– Apache Velocity: the template engine framework used to reference objects defined in Java code (a viable alternative to Java Server Pages)
I’ve reused previous articles in which I have alredy explained how to configure the Apache Velocity template engine and the persistence layer based on the Hibernate framework. The tutorial covers the creation of a demo web project called “sample-struts” in the Eclipse IDE . The sample-struts project implements the MVC pattern for a hypotetichal Customer business domain.
ROADMAP
STEP 1 – Create a web project in Eclipse, configure Struts-MVC and Velocity
STEP 2 – Basic configuration of Hibernate framework whitin the web project
STEP 3 – Enhance of Hbernate, the DAO-Factory design pattern
STEP 4 – Java POJO and Hibernate mapping
STEP 5 – CRUD functionality
STEP 1 – Create a web project in Eclipse, configure Struts-MVC and Velocity
Create a struts-blank web project in Eclipse and configure the Velocity engine. To do this follow this article (I’ve called the web project as “sample-struts” ). If no errors occur you can see the Velocity test page:
Add now a default welcome page to map the base web context http://localhost:8080/sample-struts. You can see also a link to a Customer list, the hypotethical object representing the business model layer
– WebContent/pages/index.vm
<body> <p>WELCOME! This is a Struts-Hibernate-Velocity MVC demo</p> <br> <div> <a href="./customers.do?action=multiRetrieve">--> Go to Customer List</a> </div> </body>
Define the welcome page in WebContent/WEB-INF/web.xml
<?xml version="1.0" encoding="UTF-8"?> <web-app xmlns:xsi="http://www.w3.org/2001/XMLSchema-instance" xmlns="http://java.sun.com/xml/ns/javaee" xmlns:web="http://java.sun.com/xml/ns/javaee/web-app_2_5.xsd" xsi:schemaLocation="http://java.sun.com/xml/ns/javaee http://java.sun.com/xml/ns/javaee/web-app_2_5.xsd" id="WebApp_ID" version="2.5"> <display-name>sample-struts</display-name> <welcome-file-list> <welcome-file>pages/index.vm</welcome-file> </welcome-file-list> <!-- Something other here ...--> </web-app>
Here the output of the welcome page on http://localhost:8080/sample-struts
STEP 2 – Basic configuration of Hibernate framework whitin the web project
Now go to this article and add a basic configuration of the Hibernate framework whitn the web project. If no errors occur you can see the Hibernate session factory creation at Tomcat startup.
STEP 3 – Enhance of Hbernate, the DAO-Factory design pattern
We proceed with an advanced hibernate configuration integrating the DAO-Factory design pattern within the web project. To do this follow this article. Here the partial project layout.
STEP 4 – Java POJO and Hibernate mapping
Continue with the POJO object definition and the ORM Hibernate mapping. Follow this article, you should have this new project layout.
STEP 5 – CRUD functionality
Implement the CRUD functionality within the web project following this article. If no errors occur finally you can perform the retrieve, insert, update and delete actions for the Customer domain object. Here some screenshots.
– Multi Retrieve
– Single retrieve ,update and create