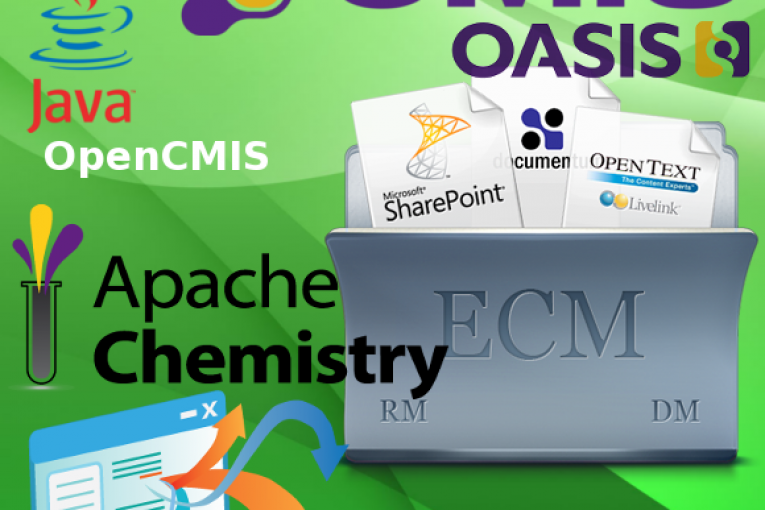
CMIS is an OASIS open standard enabling information sharing between different Content Management Systems. The CMIS standard defines a domain model and set of bindings that include Web Services and ReSTful AtomPub that can be used by applications to work with one or more ECM repositories/systems like Sharepoint, Alfresco, Nuxeo, OpenText, Documentum, IBM FileNet and so on.
Apache Chemistry, a project of the Apache Software Foundation, provides open source implementations of the CMIS specification and it allows for building applications on CMIS repositories from both the client and the server sides using a set of API libraries in Java, Python, PHP, .NET and Objective-C. In this article I want to use the Apache Chemistry OpenCMIS Client API to access the Alfresco CMIS-compliant content repository from Java code. The class diagram below shows the high level structure of the OpenCMIS Client API.
Project skeleton using Maven quickstart archetype
mvn archetype:generate -DgroupId=eu.giuseppeurso.samplecmissession -DartifactId=sample-cmis-session -DarchetypeArtifactId=maven-archetype-quickstart -DinteractiveMode=false
The OpenCMIS Client API dependency declaration in the pom.xml file
<dependency> <groupId>org.apache.chemistry.opencmis</groupId> <artifactId>chemistry-opencmis-client-impl</artifactId> <version>0.10.0</version> </dependency>
The following code snippet shows how to connect to the Alfresco repository using the AtomPub binding. The SessionFactory object, is responsible for creating of the CMIS session. I set up a parameter map that defines the credentials and connection settings for the target URL (user name, authentication, connection URL, binding type, etc.). It’s important to say that some CMIS endpoints, as such as for Alfresco, only provide one repository. In this case it is not necessary to provide its repository id. So it is sufficient get the list of all available repositories and connect to the first one invoking the method get(0).createSession(). In this example I retrieved some Alfresco Repository info over the CMIS session.
// The default factory implementation SessionFactory sessionFactory = SessionFactoryImpl.newInstance(); Map<String, String> parameter = new HashMap<String, String>(); // ECM user credentials parameter.put(SessionParameter.USER, "admin"); parameter.put(SessionParameter.PASSWORD, "pass123"); // ECM connection settings, the following ATOMPUB_URL is for Alfresco 4.0 and 4.1 parameter.put(SessionParameter.ATOMPUB_URL, "http://server:8080/alfresco/cmisatom"); parameter.put(SessionParameter.BINDING_TYPE, BindingType.ATOMPUB.value()); // Get a list of repositories retrieved by the atompub CMIS endpoint (for Alfresco there is only one element) List<Repository> repositories = sessionFactory.getRepositories(parameter); // Create CMIS session to the repository Session session = repositories.get(0).createSession(); // Get some repository info System.out.println("Repository Name: "+session.getRepositoryInfo().getName()); System.out.println("Repository ID: "+session.getRepositoryInfo().getId()); System.out.println("CMIS Version: "+session.getRepositoryInfo().getCmisVersion());
Thanks for the write-up. Just one comment that the service URL you are showing is for Alfresco 4.0. Those using Alfresco 4.2 should instead use:
http://localhost:8080/alfresco/api/-default-/public/cmis/versions/1.0/atom
OR
http://localhost:8080/alfresco/api/-default-/public/cmis/versions/1.1/atom
Depending on whether they want CMIS 1.0 or CMIS 1.1.
Thanks for your comment Jeff, I updated the code snippet.
Version of Alfresco is irrelevant here, I had Alfresco 4.1 installed and ready-to-use on my computer, so I used the first environment I had in my hands.
Anyway this article is only a quick start to introduce the CMIS Session topic, the next step is to try handling a CMIS Session. I would like to implement a CMIS authentication manager for Alfresco using Spring HandlerInterceptor. The offcial wiki of Apache Chemistry says that openCMIS Session is thread-safe. This given me a great deal to think about.
Here is what I mean with ThreadLocal, Spring MVC and HandlerInterceptor:
http://www.giuseppeurso.eu/en/alfresco-check-cmis-session-using-spring-interceptor/